Assets in Nominal with Python
To use this guide, install the Nominal Python library with pip3 install nominal
.
See Quickstart for more details.
Please contact us if you’re not sure whether your organization has access to Nominal.
An Asset is Nominal’s primitive for test data without a time domain. This guide details common patterns for working with Assets in Python.
Connect to Nominal
To instantiate an Asset, first connect to your Nominal platform tenant.
Get your Nominal API token from your User settings page.
See the Quickstart for more details on connecting to Nominal from Python.
Create an Asset
It’s possible to create an empty asset without any data.
Add data to an asset
To add a Dataset to an Asset, use Asset.add_dataset()
:
Datasets are the file representation of Nominal’s Data Source primitive. Most often, Datasets are tabular files with at least one time dimension. Datasets can also be video files.
Head over to the Datasets page to see your organization’s most recently uploaded Datasets.
Asset data with data scope names
To add a Dataset with a data scope name to an Asset, set the data_scope_name
parameter in Asset.add_dataset()
.
Data scope names are a namespace for data sources that share common channels, but do not necessarily belong to the same Asset. They allow data sources with similar schema to be referenced as a group. For example, data sources with the same data scope name can share Workbook templates and Checklists.
Check if an Asset exists
You can check for a Asset’s existence with nm.search_assets().
Update an Asset
Asset metadata can be updated with Asset.update()
:
For example, to set an Asset’s title:
Please see Asset.update()
for all updatable metadata.
Asset attachments
File attachments such as PDF reports or PowerPoints can be added to Assets:
Retrieve an Asset
Like Datasets, Assets can be retrieved by their resource ID (“RID”):
To retrieve an Asset’s RID, visit its detail page and click on the clipboard icon next to “ID” in the right-hand drawer:
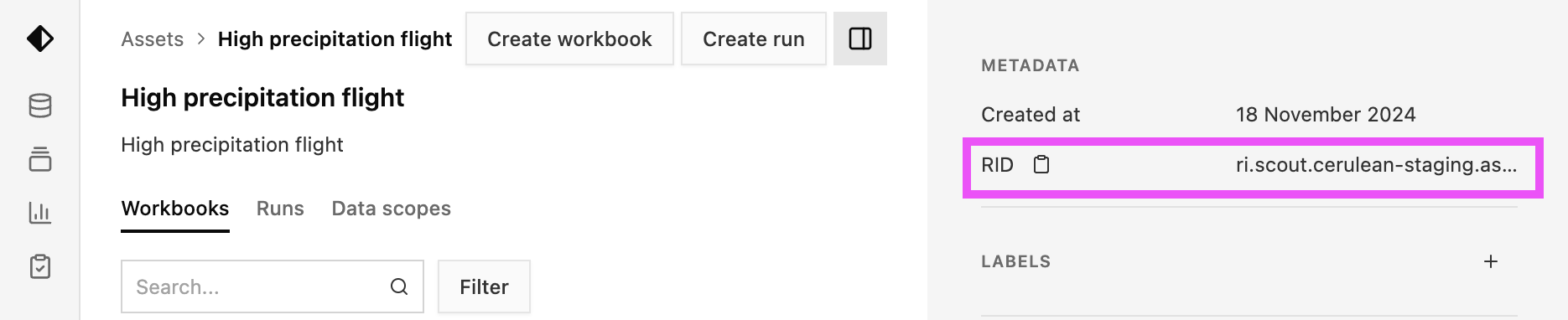
All Nominal primitives (eg Datasets, Runs, Workbooks, and Checks) have a unique identifier called a “Resource ID”.
Resource IDs may be referred to as “RID” or simply “ID” throughout the platform. They can be obtained from
a primitive’s detail page (or URL) and have a format that looks like ri.catalog.cerulean-staging.dataset.e5ede17b-05f9-404d-aaf5-ba85c99761a2
.
Query Assets
Assets can be queried with nm.search_assets().
For example, to retrieve all assets with the label “NEW-MOTOR-VENDOR”:
See nm.search_assets() for all Assets search parameters.
Remove Asset Data Sources
The list data_sources
can contain Connection, Dataset, Video instances, or rids as string.